Js Server Not Recognized Continuing With Build
Learn how you can fix JavaScript ReferenceError: require is not defined. Browser and Node.js environment
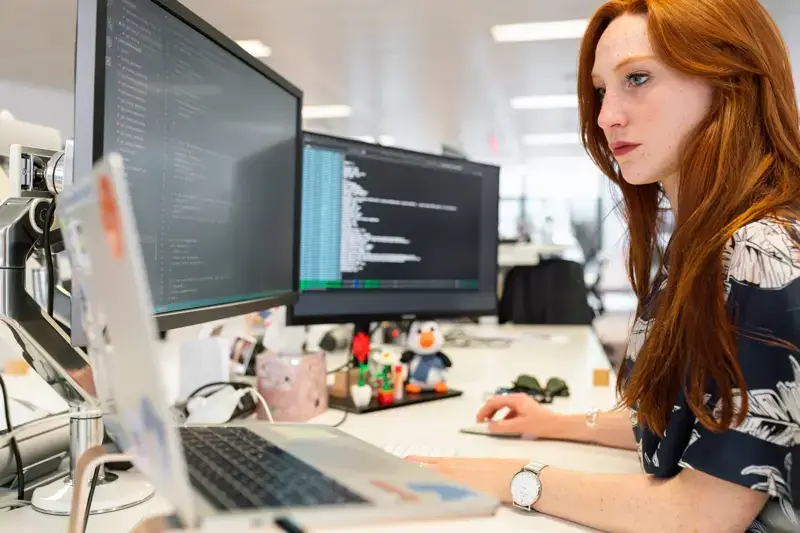
Sometimes, JavaScript may suddenly give you a require is not defined error when importing a module as follows:
const axios = require ( "axios" ); // 👇 error Uncaught ReferenceError : require is not defined
This usually happens because your JavaScript environment doesn't understand how to handle the call to require()
function you defined in your code. Here are some known causes for this error:
- Using
require()
in a browser without RequireJS - Using
require()
in Node.js withtype
:module
defined in yourpackage.json
file - Your JavaScript file has
.mjs
extension instead of.js
This tutorial will help you solve this error.
Let's see how to fix the error from the browser first.
Fix require is not defined in a browser
The JavaScript require()
function is only available by default in Node.js environment.
This means the browser won't know what you mean with the require()
call in your code.
But require()
is actually not needed because browsers automatically load all the <script>
files you added to your HTML file.
For example, suppose you have a lib.js
file with the following code:
function hello () { alert ( "Hello World!" ); }
Then you add it to your HTML file as follows:
< body > <!-- 👇 add a script --> < script src = "lib.js" ></ script > </ body >
The function hello()
is already loaded just like that. You can call the hello()
function anytime after the <script>
tag above.
Here's an example:
< body > < script src = "lib.js" ></ script > <!-- 👇 call the lib.js function --> < script > hello (); </ script > </ body >
A browser load all your <script>
tags from top to bottom.
After a <script>
tag has been loaded, you can call its code from anywhere outside of it.
Server-side environments like Node doesn't have the <script>
tag, so it needs the require()
function.
Use ESM import/ export syntax
If you need a require-like syntax, then you can use the ESM import/export syntax from a browser environment.
Modern browsers like Chrome, Safari, and Firefox support import/export syntax inside a script with type module
.
First, you need to create exports in your script. Suppose you have a helper.js
file with an exported function as follows:
function greetings () { alert ( "Using ESM import/export syntax" ); } export { greetings };
The exported function can then be imported into another script.
Create an HTML file and load the script. Add the type
attribute as shown below:
<!DOCTYPE html> < html lang = "en" > < head > < meta charset = "UTF-8" /> </ head > < body > <!-- 👇 don't forget the type="module" attribute --> < script type = "module" src = "helper.js" ></ script > < script type = "module" src = "process.js" ></ script > </ body > </ html >
In the process.js
file, you can import the helper.js
file as shown below:
import { greetings } from "./helper.js" ; greetings ();
You should see an alert box called from the process.js
file.
You can also use the import
statement right in the HTML file like this:
<!DOCTYPE html> < html lang = "en" > < head > < meta charset = "UTF-8" /> </ head > < body > < script type = "module" src = "helper.js" ></ script > <!-- 👇 import here --> < script type = "module" > import { greetings } from "./helper.js" ; greetings (); </ script > </ body > </ html >
Using RequireJS on your HTML file.
If you want to use the require()
function in a browser, then you need to add RequireJS to your script.
RequireJS is a module loader library for in-browser use. To add it to your project, you need to download the latest RequireJS release and put it in your scripts/
folder.
Next, you need to call the script on your main HTML header as follows:
<!DOCTYPE html> < html > < head > < title >RequireJS Tutorial</ title > < script data-main = "scripts/app" src = "scripts/require.js" ></ script > </ head > < body > < h1 id = "header" >My Sample Project</ h1 > </ body > </ html >
The data-main
attribute is a special attribute that's used by RequireJS to load a specific script right after RequireJS
is loaded. In the case of above, scripts/app.js
file will be loaded.
Inside of app.js
, you can load any scripts you need to use in your project.
Suppose you need to include the Lodash library in your file. You first need to download the script from the website, then include the lodash.js
script in the scripts/
folder.
Your project structure should look as follows:
├── index.html └── scripts ├── app.js ├── lodash.js └── require.js
Now all you need to do is use requirejs
function to load lodash
, then pass it to the callback function.
Take a look at the following example:
requirejs ([ "lodash" ], function ( lodash ) { const headerEl = document . getElementById ( "header" ); headerEl . textContent = lodash . upperCase ( "hello world" ); });
In the code above, RequireJS will load lodash
library.
Once its loaded, the <h1>
element will be selected, and the textContent
will be replaced with "hello world" text, transformed to uppercase by lodash.uppercase()
call.
You can wait until the whole DOM is loaded before loading scripts by listening to DOMContentLoaded
event as follows:
document . addEventListener ( "DOMContentLoaded" , function () { requirejs ([ "lodash" ], function ( lodash ) { const headerEl = document . getElementById ( "header" ); headerEl . textContent = lodash . upperCase ( "hello world" ); }); });
Finally, you may also remove the data-main
attribute and add the <script>
tag right at the end of the <body>
tag as follows:
<!DOCTYPE html> < html > < head > < title >RequireJS Tutorial</ title > < script src = "scripts/require.js" ></ script > </ head > < body > < h1 id = "header" >My Sample Project</ h1 > < script > document . addEventListener ( "DOMContentLoaded" , function () { requirejs ([ "scripts/lodash" ], function ( lodash ) { const headerEl = document . getElementById ( "header" ); headerEl . textContent = lodash . upperCase ( "hello world" ); }); }); </ script > </ body > </ html >
Feel free to restructure your script to meet your project requirements.
You can download an example code on this requirejs-starter repository at GitHub.
Now you've learned how to use RequireJS in a browser. Personally, I think it's far easier to use ESM import/export syntax because popular browsers already support it by default.
Next, let's see how to fix the error on the server-side
Fix require is not defined on server-side
The require()
function is used when you need to load a package or a module into your JavaScript file.
For example, here's how to load lodash
from Node:
// 👇 load library from node_modules const lodash = require ( "lodash" ); // 👇 load your exported modules const { greetings } = require ( "./helper" );
But even when you are running the code using Node, you may still see the require is not defined error because of your configurations.
Here's the error logged on the console:
$ node index.js file:///DEV/n-app/index.js:1 const { greetings } = require( "./helper" ) ; ^ ReferenceError: require is not defined
If this happens to you, then the first thing to do is check your package.json
file.
See if you have a type: module defined in your JSON file as shown below:
{ "name" : "n-app" , "version" : "1.0.0" , "type" : "module" }
The module
type is used to make Node treat .js
files as ES modules. Instead of require()
, you need to use the import/export syntax.
To solve the issue, remove the "type": "module"
from your package.json
file.
If you still see the error, then make sure that you are using .js
extension for your JavaScript files.
Node supports two JavaScript extensions: .mjs
and .cjs
extensions.
These extensions cause Node to run a file as either ES Module or CommonJS Module.
When using the .mjs
extension, Node will not be able to load the module using require()
. This is why you need to make sure you are using .js
extension.
Conclusion
And now you've learned the solutions to the ReferenceError: require is not defined
issue from the server and browser environment.
I've also written several other common JavaScript errors and how to fix them:
- How to fix JavaScript unexpected token error
- How to fix JavaScript function is not defined error
- Fixing JavaScript runtime error: $ is undefined
These articles will help you become better at debugging JavaScript issues.
Thanks for reading! 🙏
Source: https://sebhastian.com/javascript-require-is-not-defined/